Description
This project is an API which returns the list of articles from my website hajereducation.tn
You can see the list of the latests articles from all the categories on my website or the list of the latests articles from a specific category .
Environment : Code editor , js , …
- VS Code
- Windows 10
- node js
- express
- axios
- cheerio
- git & github
- heroku
- RapidAPI
Axios
Axios is a promise-based HTTP Client for node.js
and the browser. It is isomorphic (= it can run in the browser and nodejs with the same codebase). On the server-side it uses the native node.js http
module, while on the client (browser) it uses XMLHttpRequests.
cheerio
Cheerio parses markup and provides an API for traversing/manipulating the resulting data structure. It does not interpret the result as a web browser does. Specifically, it does not produce a visual rendering, apply CSS, load external resources, or execute JavaScript which is common for a SPA (single page application). This makes Cheerio much, much faster than other solutions. If your use case requires any of this functionality, you should consider browser automation software like Puppeteer and Playwright or DOM emulation projects like JSDom.
Steps
- create new Repository Github
- Code –> SSH –>Copy
- git clone SSH
git clone git@github.com:hajer77/hajereducation-api.git
cd hajereducation-api
code .
npm init
- Results npm init
{ "name": "hajereducation-api", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "repository": { "type": "git", "url": "git+https://github.com/hajer77/hajereducation-api.git" }, "author": "Hajer Dahech", "license": "ISC", "bugs": { "url": "https://github.com/hajer77/hajereducation-api/issues" }, "homepage": "https://github.com/hajer77/hajereducation-api#readme" }
- Create new file index.js
- Install cheerio
npm i cheerio
- Package.json
“dependencies”: {
“cheerio”: “^1.0.0-rc.12”
}
{ "name": "hajereducation-api", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "repository": { "type": "git", "url": "git+https://github.com/hajer77/hajereducation-api.git" }, "author": "Hajer Dahech", "license": "ISC", "bugs": { "url": "https://github.com/hajer77/hajereducation-api/issues" }, "homepage": "https://github.com/hajer77/hajereducation-api#readme", "dependencies": { "cheerio": "^1.0.0-rc.12" } }
- Install express.js
npm i express
- Results Package.json
“dependencies”: {
“cheerio”: “^1.0.0-rc.12”,
“express”: “^4.18.1”
}
{ "name": "hajereducation-api", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "repository": { "type": "git", "url": "git+https://github.com/hajer77/hajereducation-api.git" }, "author": "Hajer Dahech", "license": "ISC", "bugs": { "url": "https://github.com/hajer77/hajereducation-api/issues" }, "homepage": "https://github.com/hajer77/hajereducation-api#readme", "dependencies": { "cheerio": "^1.0.0-rc.12", "express": "^4.18.1" } }
- install axios
“dependencies”: {
“axios”: “^0.27.2”,
“cheerio”: “^1.0.0-rc.12”,
“express”: “^4.18.1”
}
{ "name": "hajereducation-api", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "repository": { "type": "git", "url": "git+https://github.com/hajer77/hajereducation-api.git" }, "author": "Hajer Dahech", "license": "ISC", "bugs": { "url": "https://github.com/hajer77/hajereducation-api/issues" }, "homepage": "https://github.com/hajer77/hajereducation-api#readme", "dependencies": { "axios": "^0.27.2", "cheerio": "^1.0.0-rc.12", "express": "^4.18.1" } }
git add .
git commit -m "add files"
git push origin HEAD
- index.js
const express = require('express'); const axios = require ('axios'); const cheerio = require('cheerio'); const app = express(); const port = process.env.PORT || 3000; const articles = []; const hajereducationCategories=[ { category:'python', address:'https://hajereducation.tn/category/python-3-7/' }, { category:'netbeans', address:'https://hajereducation.tn/category/netbeans-tutorial/' }, { category:'matlab', address:'https://hajereducation.tn/category/matlab/' }, { category:'embeddedSystem', address:'https://hajereducation.tn/category/embedded-system-projects/' }, { category:'chimie', address:'https://hajereducation.tn/category/chimie/' }, { category:'physique', address:'https://hajereducation.tn/category/physique/' }, { category:'math', address:'https://hajereducation.tn/category/mathematiques/' }, { category:'coding', address:'https://hajereducation.tn/category/coding/' }, { category:'bac', address:'https://hajereducation.tn/category/bac/' }, { category:'1ereAnnee', address:'https://hajereducation.tn/category/1ere-annee-%d8%a3%d9%88%d9%84%d9%89-%d8%ab%d8%a7%d9%86%d9%88%d9%8a/' }, { category:'2emeAnnee', address:'https://hajereducation.tn/category/2eme-annee-secondaire/' }, { category:'informatique', address:'https://hajereducation.tn/category/informatique/' }, { category:'manuelScolaire', address:'https://hajereducation.tn/category/manuel-scolaire/' } ]; hajereducationCategories.forEach(hajereducationCategory=>{ axios.get(hajereducationCategory.address) .then((response)=>{ const html=response.data; //console.log(html); const $=cheerio.load(html); $('.post-card__container' , html).each(function(){ const title =$('h2',this).text(); const url = $('a',this).attr('href'); articles.push({ category :hajereducationCategory.category, title, url }) }) }).catch(err => console.error(err)); }) app.get('/',(req,res)=>{ res.json('My website hajereducation.tn API'); }) app.get('/hajereducation',(req,res)=>{ res.json(articles); }) app.get('/hajereducation/:hajereducationCategoryId', (req,res)=>{ const hajereducationCategoryId=req.params.hajereducationCategoryId; const hajereducationCategory = hajereducationCategories.filter(hajereducationCategory=>hajereducationCategory.category == hajereducationCategoryId); const hajereducationCatAddress = hajereducationCategories.filter(hajereducationCategory=>hajereducationCategory.category == hajereducationCategoryId)[0].address; axios.get(hajereducationCatAddress) .then(response=>{ const html =response.data; const $= cheerio.load(html); const categoryArticles =[]; $('.post-card__container' , html).each(function(){ const title =$('h2',this).text(); const url = $('a',this).attr('href'); categoryArticles.push({ Category:hajereducationCategoryId, title, url }) }) res.json(categoryArticles); }).catch(err => console.error(err)); }) app.listen(port, () => { console.log(`Example app listening on port ${port}`); })
- Github
npm i nodemon
git add .
git commit -m "add code "
git push origin HEAD
- install JSON Viewer extension Google Chrome
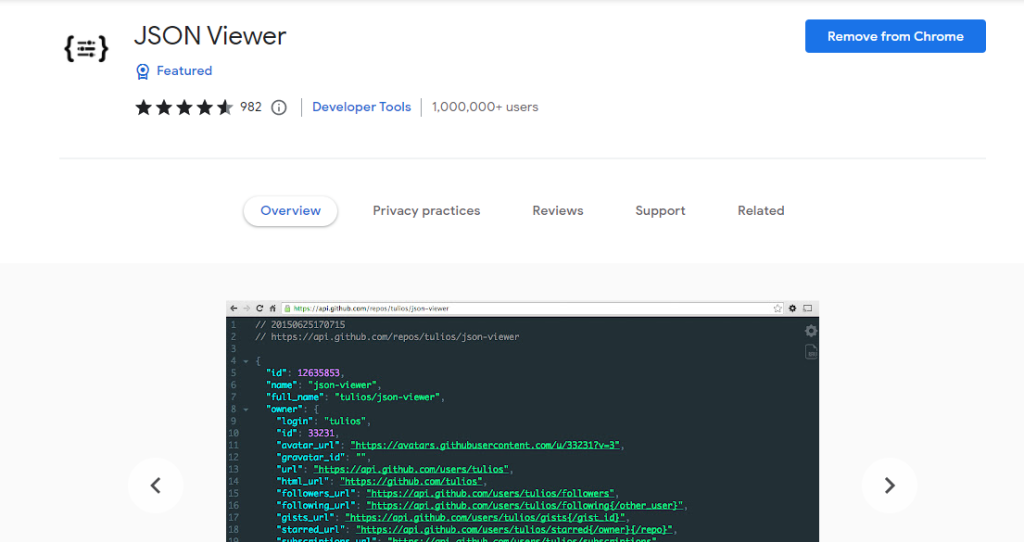
- deploy the app with heroku https://hajereducation-api.herokuapp.com/hajereducation/
heroku login
heroku git:remote -a hajereducation-api
git push heroku main
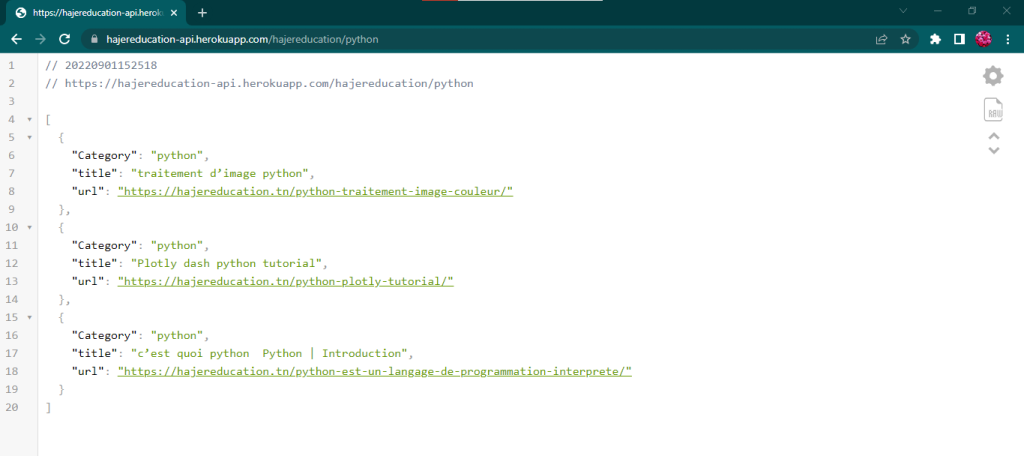
- Add API project : https://rapidapi.com/studio
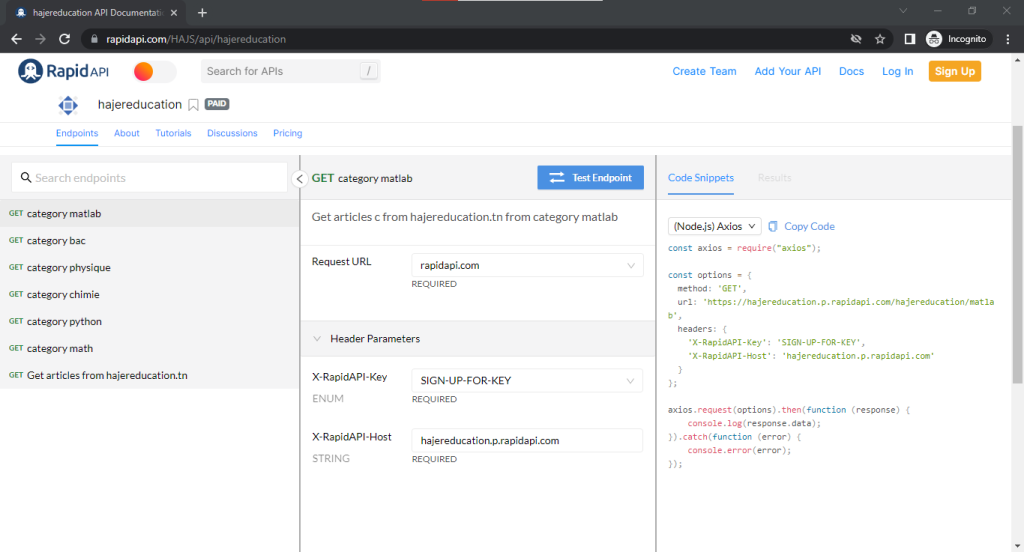
- Test Endpoint with rapidAPI
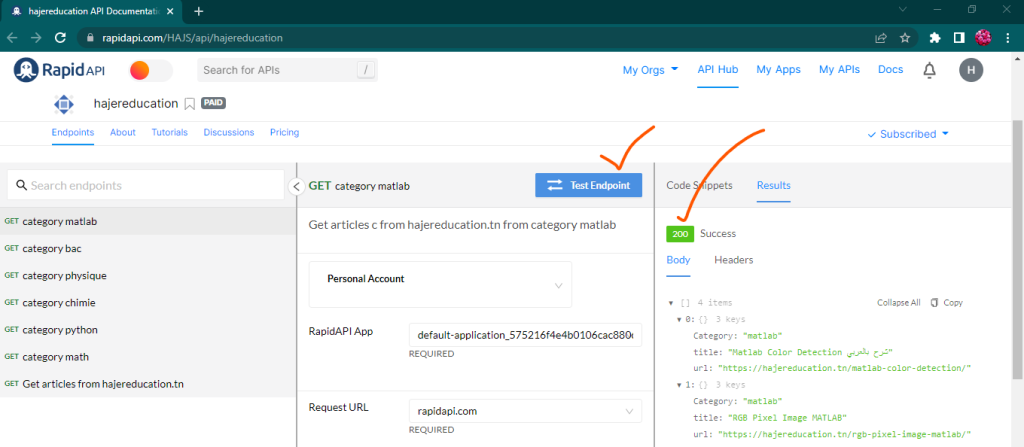
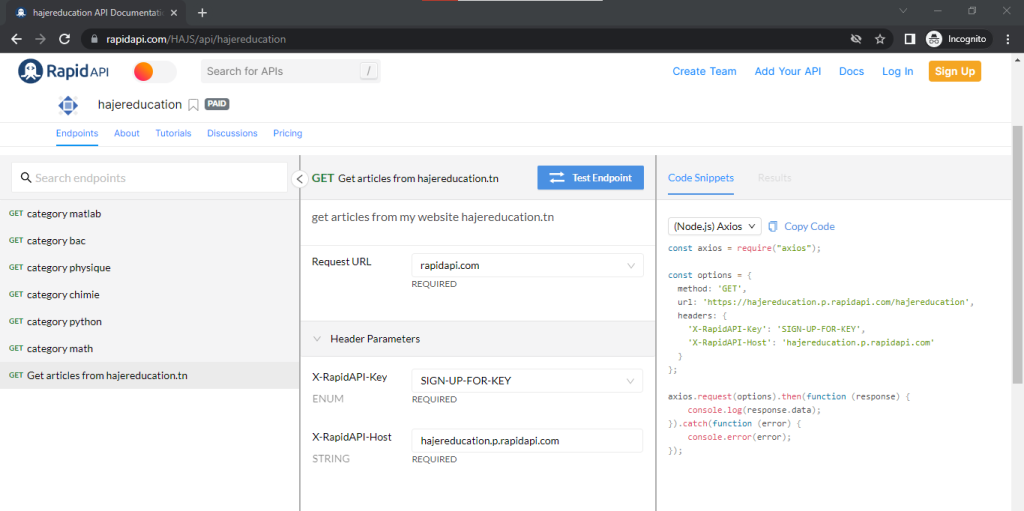